Path to React Native: From Rookie to Rockstar
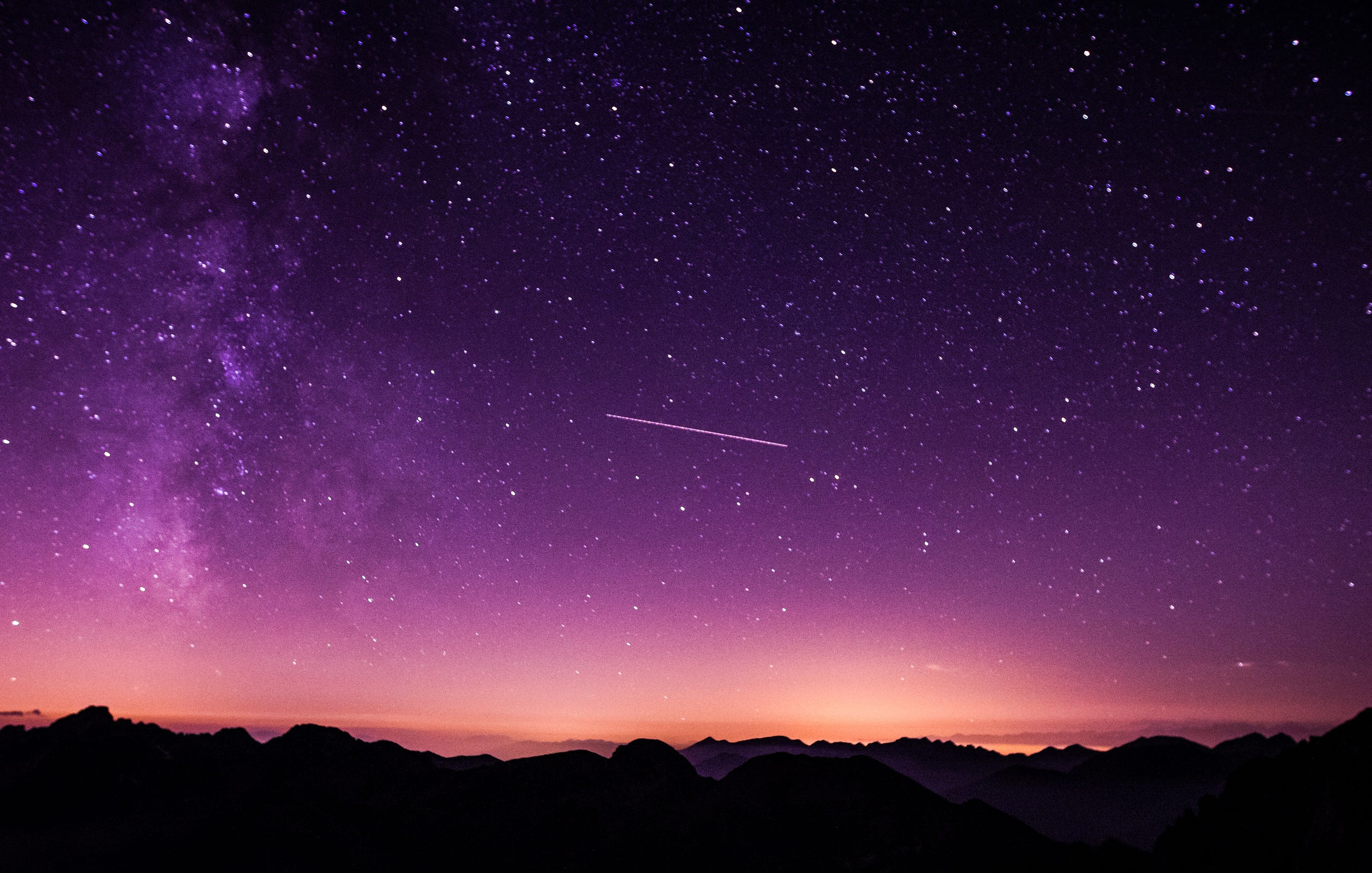
Building a hybrid mobile app seemed like a really big challenge just a few years ago. Unless you wanted to earn a reputation for building half-arsed and sluggish mobile app interfaces, you’d have been better off spending your time and money on proper (native) iOS or Android developers instead.
Hybrid apps still carry an image of being slow and sluggish. And it’s true that WebView-based frameworks such as Ionic still have a hard time keeping up with native performance. Especially when apps start to get more complex and have to handle a lot of data, transitions and interactions.
With React Native though, things have changed. We’re now able to build native apps for both iOS and Android platforms using pure JavaScript. React Native has cleverly eliminated the layer between user interface and native code with a smart component-based approach and the versatile ”Flexbox” layout system.
But that’s not only it. Since JavaScript is so easy and popular, it turns out that even some pure iOS developers are turning their back on iOS development in favor of the more clean and organized way that JavaScript apps are written and the benefits of the new React Native toolkit.
Your road to React mastery
I’ve spent the last couple of months working with React and React Native. Having worked my way towards building reactive applications, I have had to deal with a pretty lengthy learning curve and a few new technologies that needed to be mastered.
I’ve put together a list of resources for everyone who has heard the buzz about React and React Native but hasn’t yet got to grips with how to use it. This guide should help you get towards mastery a lot quicker!
The basics: ES6, or ECMAScript 2015
The first thing you should know is that all the shiny new React Native stuff is using ES6 a lot (officially called ES2015): the next version of JavaScript.
ES6 usage isn’t strictly mandatory, but there’s really no reason not to use it. This is a great opportunity to embrace this new piece of technology with minimal downsides. ES6 has many really great new features such as classes, arrow functions, the spread operator and variable restructuring, all of which makes our lives easier.
There’s no need to worry about ES6 support either. If you set up a React Native project from scratch, ES6 support is configured for you by default. And if you’re building with React.js for the web, just use the Babel JavaScript transpiler.
Get started:
- Understanding ES6: Mozilla provides an in-depth article series which serves as a great introduction to the most relevant ES6 features.
- A hands-on ES6 introduction: If you don’t enjoy reading long technical blog posts, you might enjoy the ES2015 course on CodeSchool. It’s pretty fun and includes some hands-on examples.
- The speedy read: If you’d rather take a shortcut and spend minimal time on this, opt for a quick read like James K Nelson’s ”ES6 — The bits you’ll actually use“.
React.js
After familiarizing yourself a little with the basics of the ES2015 ecosystem, you may want to jump right in and get to know the framework better by building your first app.
Learning React.js is absolutely necessary to be a strong React Native developer. It has the same API and the app structure is almost the same. You’ll also find that because of the maturity of the library, there are many more tutorials available for React.js.
There are many great ones online from some of the most experienced online teachers. I’d recommend working through at least one of these to get familiar with React.
If you’re absolutely determined to skip this step, scroll down to the ‘React Native’ section below, where you’ll find some shortcuts.
Get started:
-
Powering Up with React is a typical CodeSchool hands-on introduction to React.js. It has fun and entertaining exercises with rewards along the way. In its five levels, it covers properties and state, synthetic events and talking to remote servers. This is a great option for beginners.
-
React for Beginners by Wes Bos (the creator several very popular tutorial series). As with CodeSchool’s course, this is a fun and entertaining step-by-step video series (about 5+ hours of content) where you build a (nearly) real world React.js application with Firebase. It’s extremely beginner friendly and covers a very wide range of React fundamentals and also explains ES6, React mixins, authentication and animation. It will probably take you about 10 hours to complete if you’ve used JavaScript before, but not ES6 or React.
-
React fundamentals by egghead is a short and sweet tutorial about all the basics if you want to quickly get up to speed with React.js. It’s probably best suited for intermediate and advanced developers though.
Flexbox
For layouts, React Native implements Flexbox. It’s a great choice for laying out small-scale components, mobile applications and gives you a lot of well… flexibility. Especially when working with components that need to be sized dynamically. Perfect for mobile screens, especially on Android, where we’ll need to support many different screen sizes.
Get started:
- Watch the video tutorials: I highly recommend Wes Bos’ course “What the Flexbox?” which explains each of the flexbox parameters in detail.
- If you’re more into reading: CSS Tricks has a long and detailed guide to Flexbox.
- Experiment in your browser! Once you understand the basics, the best way to learn Flexbox is to just play with it. All you have to do is assign the property
display: flex;
to an element, and then assign it different properties for its most common properties justify-content, align-items, flex-wrap, flex-grow and flex-shrink.
React Native
Once you’re familiar with the React basics, you’re actually equipped with all the knowledge necessary to build native mobile apps for iOS and Android.
Again, it’s useful to let someone guide you and explain why certain things are done in certain ways, instead of just reading the (rather dry) documentation.
Get started:
-
Short and focused introductory videos: React Native Fundamentals on egghead. I’ve found this series to be very useful since it contains short videos with necessary details only. You’ll build a basic iOS app with the most basic components like Text, Image, TouchableHighlight and understand the basic concept of building in React Native. The rest is about retrieving data from a remote API and updating the state of your app to display that data on the screen.
-
Build Apps with React Native on Udemy is an 8-hour introduction to React Native that you can complete without needing any React.js knowledge. The author, Stephen Grider, walks you through the nuts and bolts of JSX, components and properties before jumping into React Native, Flexbox styling and some more advanced topics like fetching remote data and authentication. If you want to take a shortcut and don’t want to bother looking into React.js in detail first, then this course is probably for you.
We’ve been building apps with React and React Native for a while now and have been getting steadily more impressed with the way the ecosystem is developing. The community is strong and the resources out there are steadily improving. Why wait? React looks like it has a very bright future ahead of it.
Originally published on Medium on June 29, 2016.